Archive
Learn PowerShell in Two Hours on YouTube
Microsoft PowerShell Documentation:
https://learn.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.4
YouTube PowerShell Training:
To determine the version of PowerShell running on your machine, use the $PSversionTable command:
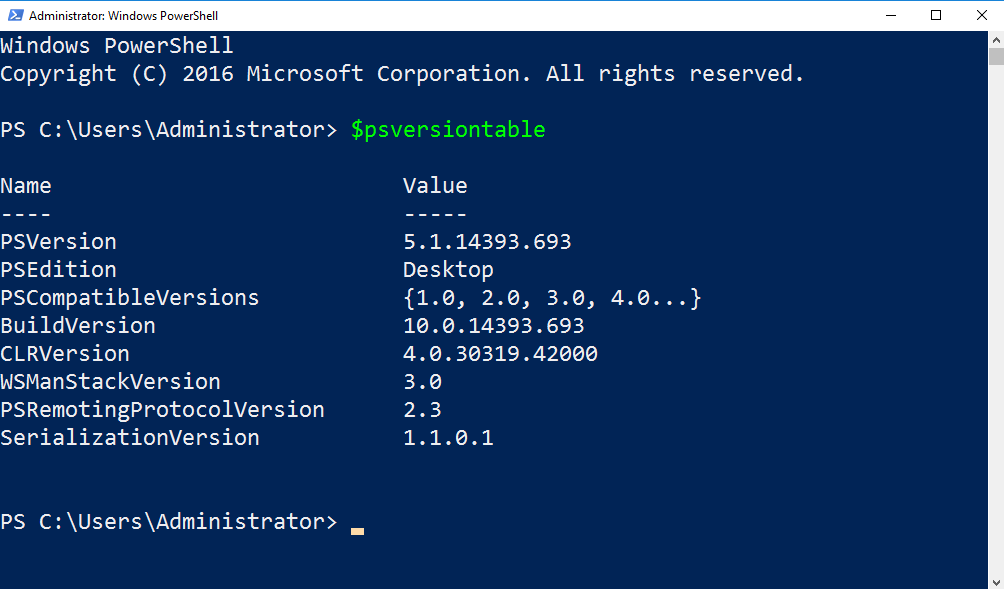
To change the version of PS (because some cmds are only valid on certain versions), issue the powershell -version [ver #] command:

Now the console is running PowerShell version 3. Keep in mind that when you close the console and reopen it, you’ll be back to whatever version is installed on the system. This is just a temporary change.
At the time of this class recording, the common version used today is PowerShell version 6:
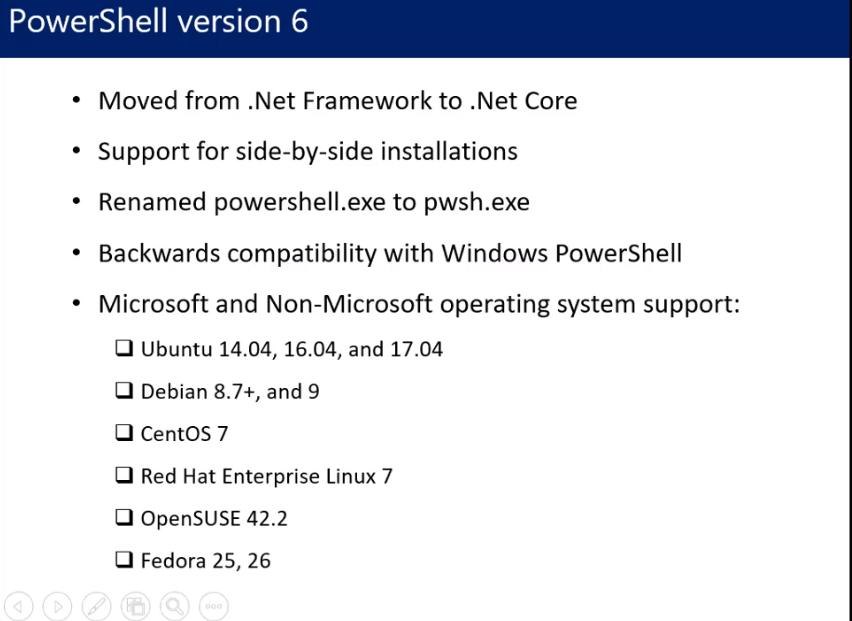
Two options for installing PS ver 6:
URL to download PowerShell Version 6
https://github.com/powershell/powershell
PowerShell command to download PowerShell Version 6
iex “& {$(irm https://aka.ms/install-powershell.ps1)} -UseMSI”
Changing the Execution Policy
By default the execution policy is set to Restricted. This means that we cannot run custom scripts (only Microsoft approved scripts). To change this:
Open PS as admin > Set-ExecutionPolicy RemoteSigned > y
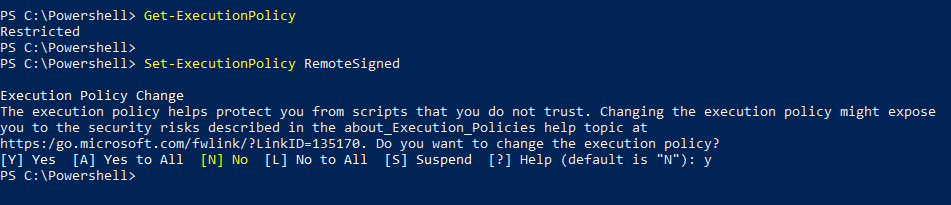
Windows PowerShell ISE
The PowerShell ISE (Integrated Scripting Environment) is a graphical user interface (GUI) tool for Windows PowerShell.
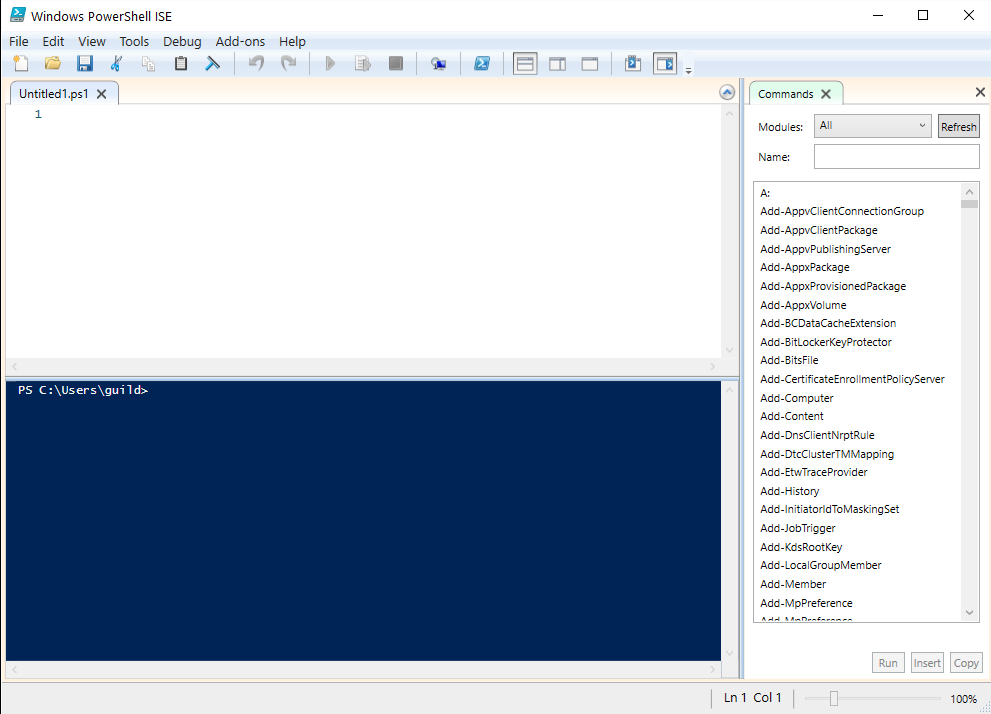
Write Your First Script
In top white window pane, type:
Write-Host “Hello World!”
Then save it in a directory on C: drive
Now run it by pressing the green right arrow on the top
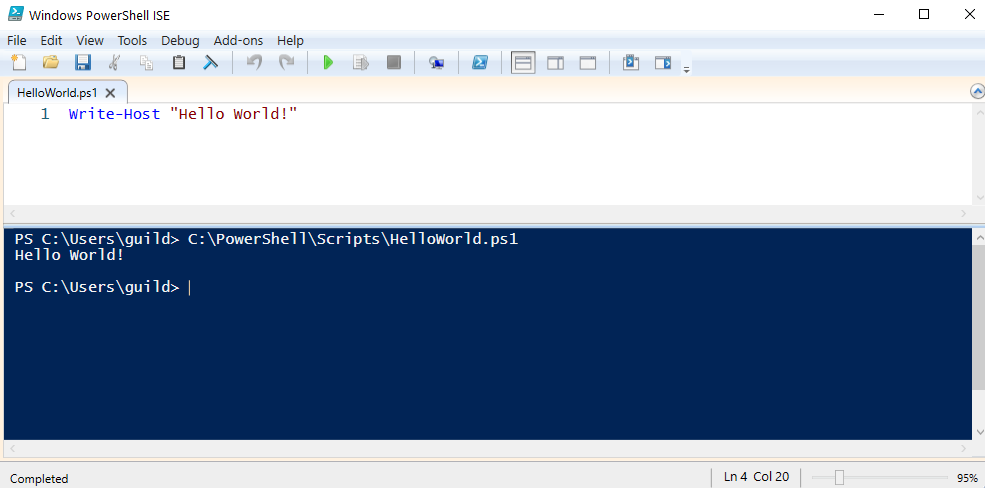
#Comments
On line one, type:
#My First Comment!
Save and run again.
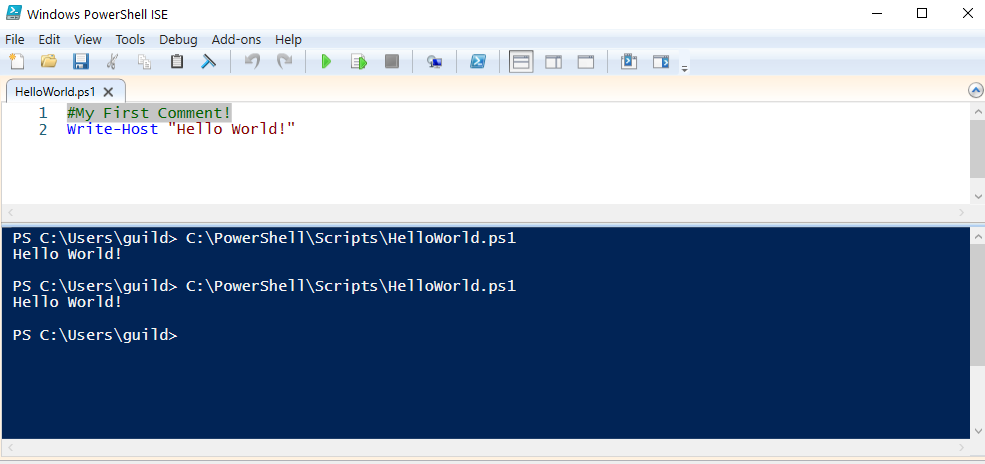
If you put a # in front of any line of code, it will not appear in the script’s output.
PowerShell consists of modules and cmdlets
Modules – a collection of settings.
Cmdlets – just the commands.
All cmds in powershell use the verb-noun syntax.
Using Write-Host is an example of a cmdlet:
On line 3 you can type Write-Host “Hello again!”
Then save and run again and it adds a new line on the output:
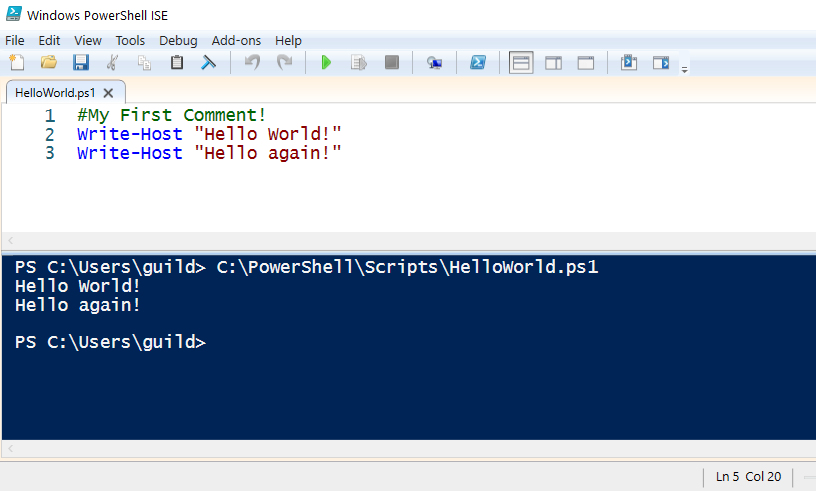
Lets say you didn’t want the Write-Host “Hello again!” on a newline. You can add a parameter that starts with –
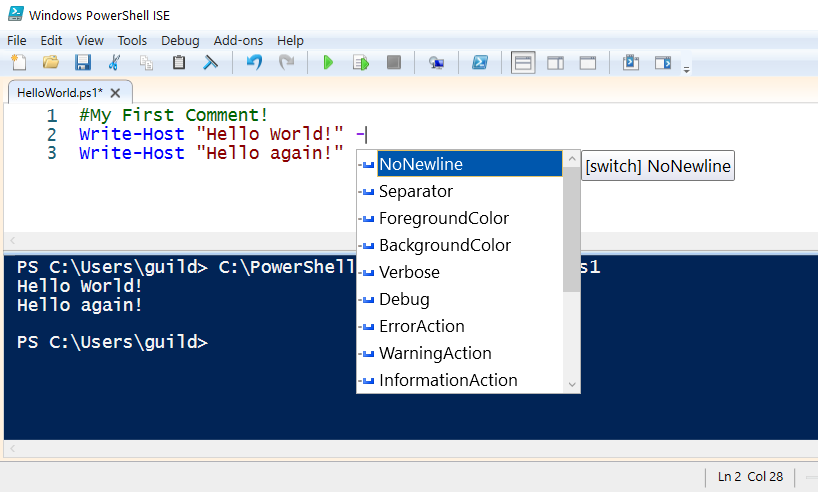
Press enter on NoNewline > save > run script:
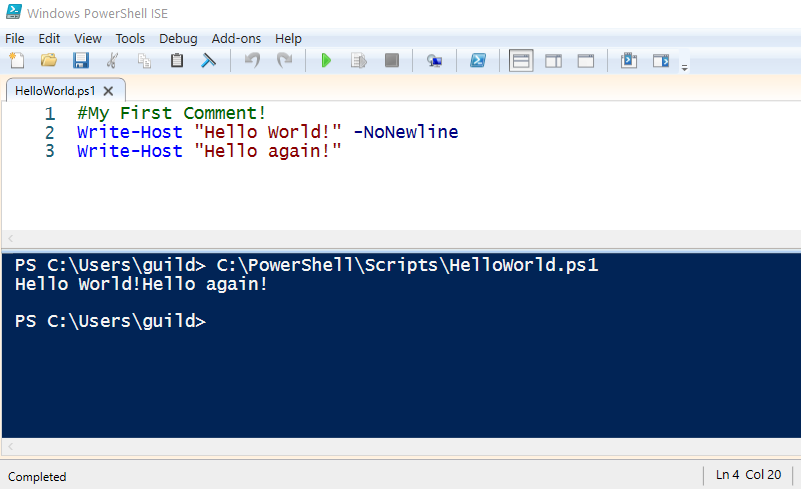
Notice the two Write-Host cmdlets are on the same line of the script output.
Finding cmdlets
Get-Command -CommandType Cmdlet
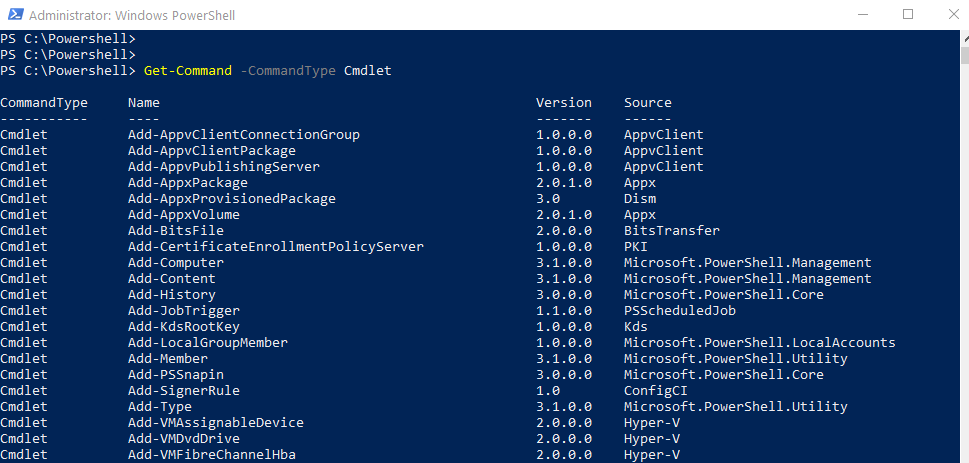
This cmd shows you all the cmdlets in your powershell.
For example: get-service or get-process
Using Pipes
With pipes, you are taking the output of one command and connecting it to another command on the other side of the pipe. For example:
AnyCommand 1 | AnyCommand 2
“AnyString” | Out-File test.txt
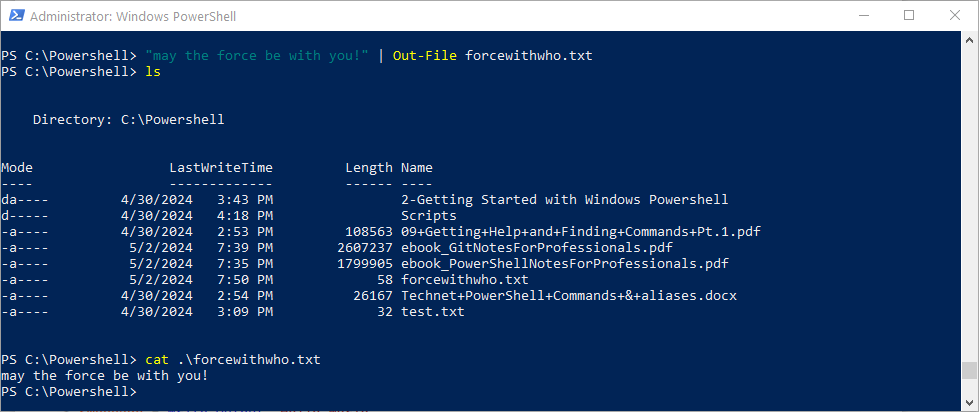
So what we did above was I wrote a random string “may the force be with you!” (must have quotes on both sides) followed by a pipe | and then use the command Out-File (which prints the string to a file) named forcewithwho.txt
That created a file in my C:\Powershell folder. Which can be displayed by using:
cat .\forcewithwho.txt
$Variables
Variables in PS are invoked using the dollar sign symbol $
For example:
$FavCharacter = “Han Solo”
That’s it. We’ve just set the variable FavCharacter to Han Solo
And if you type your variable $FavCharacter and press enter, you’ll see the string Han Solo printed to the screen:

Now let’s pipe that same variable into a new file like we did previously:
$FavCharacter | Out-File favcharacter.txt
Do another ls command and you’ll see the new file favcharacter.txt
Next issue cat .\favcharacter.txt and you’ll see the txt we assigned to the variable print out on the screen from the file we just created:
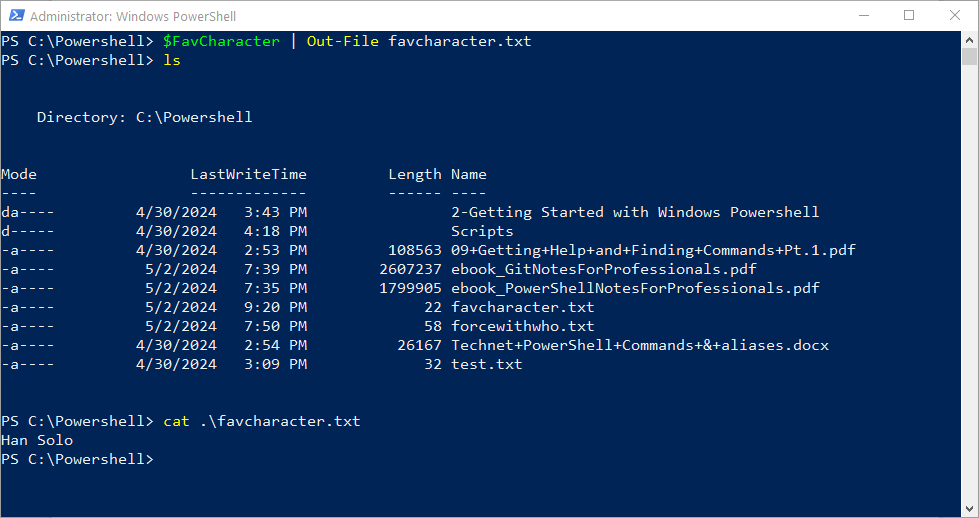
Note: For the cat .\favcharacter.txt command above, you can just type cat f and then press tab and it will autocomplete for you.
Common Data Types
Strings – a group of characters placed between double quotes (or single quotes). For example “Hello World” or ‘Hello World’
Integers – Whole numbers. For example 3 or 10
Floating Points – decimal numbers. For example .4 or .9
Booleans – True of False
To determine the data type of any variable, use the .GetType() command after your variable:
$FavCharacter.GetType()
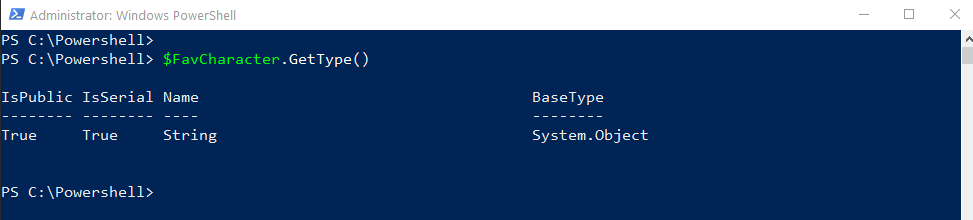
PowerShell tells us that it is a string.
We could change our variable to an integer or a boolean:
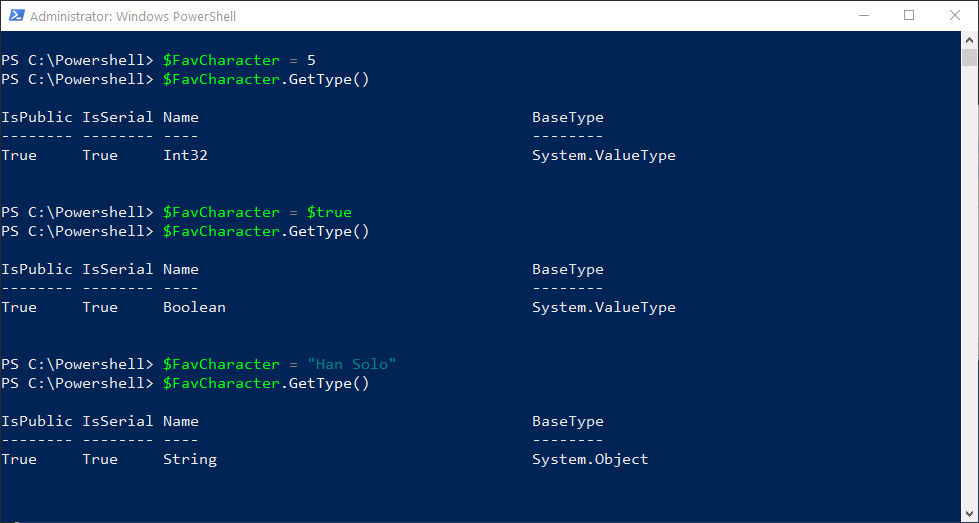
The last command I changed our variable back to the string Han Solo.
We can also do arithmetic using variables as shown below:
PS C:\Powershell> $x = 5
PS C:\Powershell> $y = 3
PS C:\Powershell> $x + $y
8
PS C:\Powershell> $x * $y
15
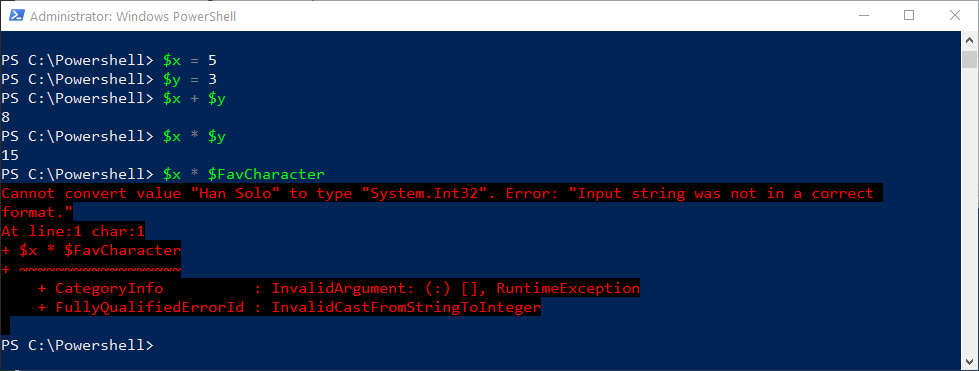
Notice above if we try mix data types by multiplying $x by $FavCharacter we get a red error message.
Objects
In PowerShell, an object is a collection of data that represents an item. Our variable $FavCharacter is actually an object.
Object Properties
Objects have properties that store info about the object. For example:
$FavCharacter.Length
The only property for our string variable is the .length which gives us how many characters are in the object. But if we had a different more complex object, you can see all the properties associated with it by:
object | Select-Object -Property *
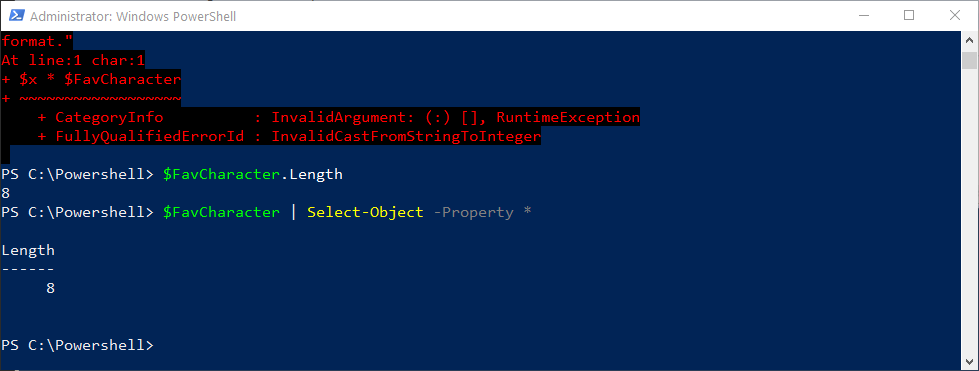
Notice only our Length property shows up since our Object(variable) is a simple string.
Object Methods
The object methods are actions that you can perform on the object.
To get all the methods for a given object issue:
Get-Member -InputObject $FavCharacter
and you’ll get a long list of methods you can perform on the object. We actually used one earlier with the GetType to determine our data type:
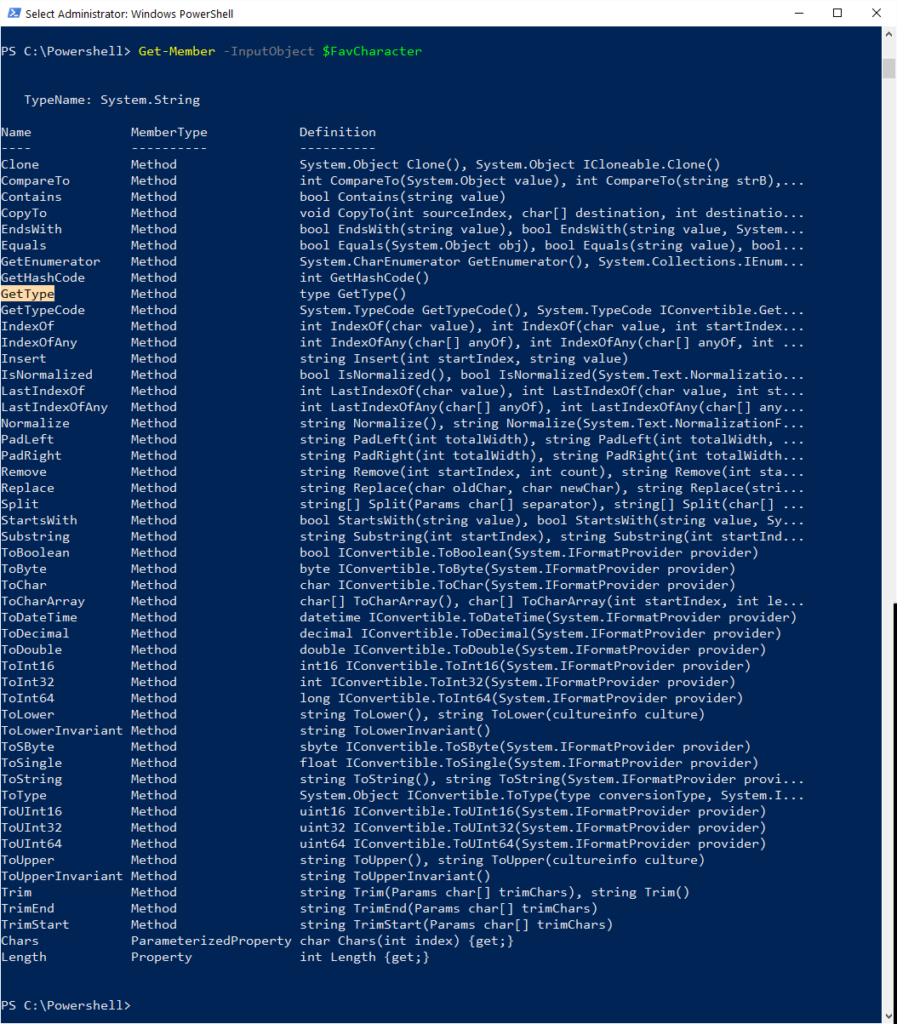
Arrays
In PowerShell, arrays are data structures designed to store a collection of items. These items can be of the same type or different types. Arrays are a fundamental part of PowerShell, or any programming language, because they allow you to store and structure a collection of items into a single variable.
The values stored in the array are delimited with a comma:
$Vikings = @(‘Ragnar Lothbrok’, ‘Bjorn Ironside’, ‘Ivar the Boneless’)
So we just set an array into a variable named Vikings with three strings inside of the array.
If we wanted to see what is in the array, just type:
$Vikings
PowerShell will print out the array:
Ragnar Lothbrok
Bjorn Ironside
Ivar the Boneless
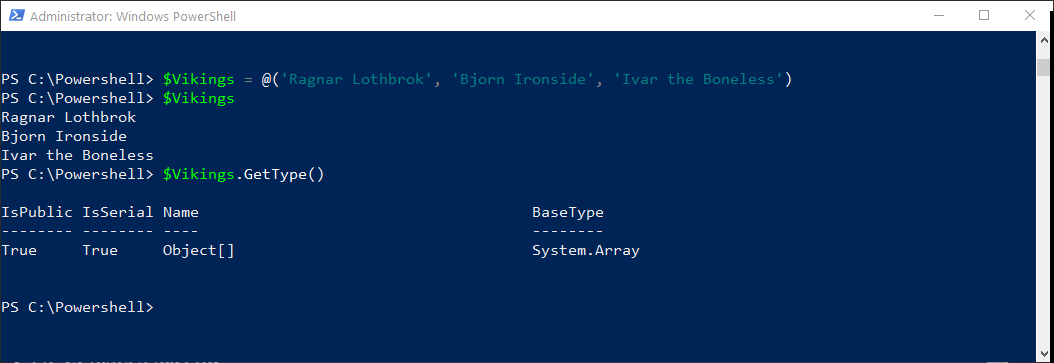
Note above we did a $Vikings.GetType() and under BaseType it says System.Array
If we wanted to access a single item in the array we would use brackets [ ] with an offset value starting at 0. For example:
To get the first item in an array (Ragnar Lothbrok):
$Vikings[0]
To get the second item (Bjorn Ironside):
$Vikings[1]
To get the third item (Ivar the Boneless):
$Vikings[2]
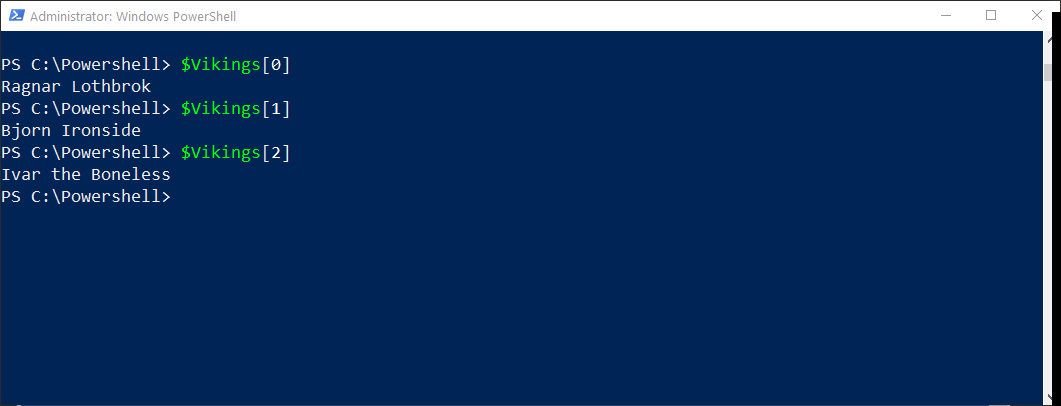
We can also use the property .length to get the character length for each:
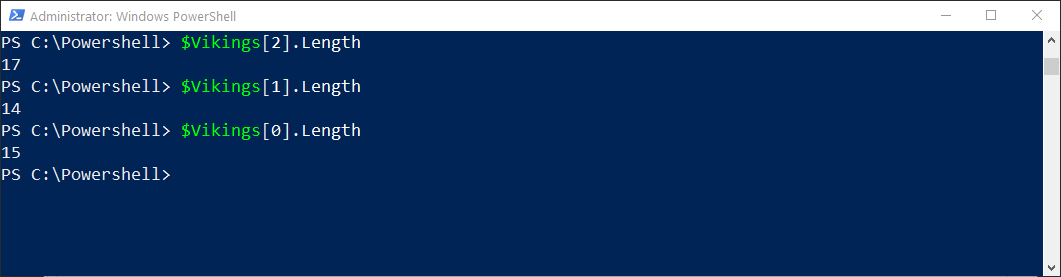
To add an item to the array, call the variable and use += followed by the new item:
$Vikings += ‘Leif Ericson’
Call the array again to see the addition:
PS C:\Powershell> $Vikings
Ragnar Lothbrok
Bjorn Ironside
Ivar the Boneless
Leif Ericson
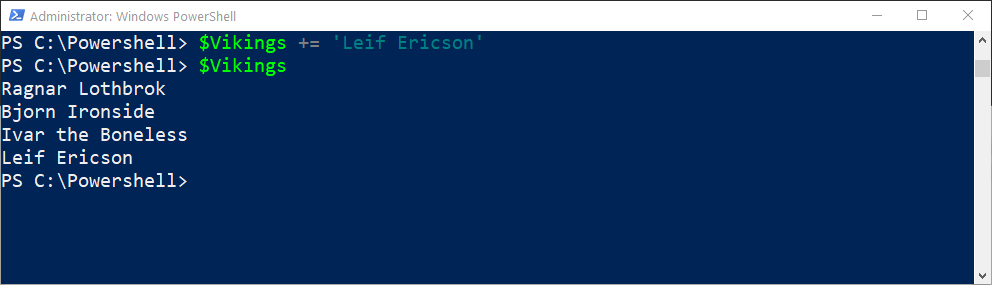